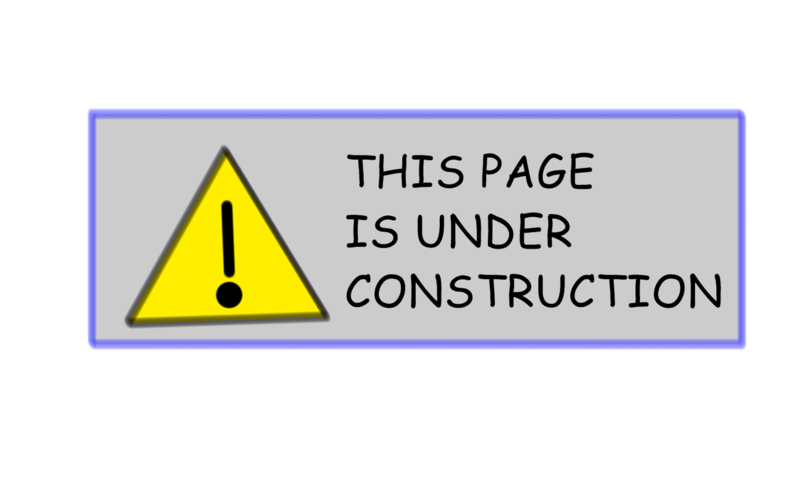
1. Input handling
This sequence of exercises focuses on recurrent issues to be considered when dealing with input, and goals in handling them: how to store the input in memory, where to retrieve it from, how to identify its end, whether it comes in a format directly ready for processing or not.Students should focus on the following aspects related to the input, which come up constantly in the design of programs:
Organization. Are the data to be processed “sparse data”, that is separate, each with a distinct name, or “data in series” that can be uniformly processed?
Memory. How many and which data is it necessary to have in memory at a time? If they are a series, do we need the whole series or just the last read value, or the last two (or a fixed number of values)? The data are already in memory (as for os.Args
) or do we need to store them?
Type. What type are the data? And how should they be stored? In one or more separate variables or rather in data structures and which ones (string, array, map, etc.)?
Readiness. Are the data ready to be processed or is some kind of preprocessing (compute, extract, etc.) needed? For example, if we stored a number but have to process its digits, we will need to extract the digits from the number; if we stored a line of text in a string, but want to process its words, we will need to identify the words in the string.
Channel/Source How are the data received? From standard input, from the command line, from a file, as returned values from a function, by a function through its parameters?
Halting. In reading our data, when do we have to stop? Do we have to read a known number of data? read until a sentinel value? or until a condition is verified or an event occurs? Do we have to read “complex” data (a string of characters, a line of text, a text file, …) as a whole or by its parts (by characters, by strings, by lines, …)?
The first 5 exercises present program comprehension tasks that focus on how the input is handled and why.
Type: Program analysis
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. In particular the focus here is whether storing the series of input numbers is necessary or not and, if necessary, how to store it.
Text: in English, in Italian
Text attachment: last_occurrence.go
Type: Program analysis
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. The focus here is whether the input data are ready to be processed or must be first preprocessed in order to compute/extract other data. For example, a string is both a value and a collection of values (characters) that can be acquired with a sigle reading. If the single characters are to be processed, as in this case, the string has to be preprocessed in order to extract them.
Text: in English
Text attachment: capitalize.go
Type: Program analysis and program addition/tailoring
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. The focus here is the use of a sentinel value to stop the reading and whether storing the series of input numbers is necessary or not and how this depends on the requests. In particular for version 1 of the program this is not necessary and for version 2 it is.
Text: in Italian only
Text attachment: rainfall.go
Type: Program analysis and program tailoring
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. The focus here is the use of the command line for passing data to a program and what this implies: that the data are strings, that they are automatically saved in a structure (a slice in Go), and that they might need to be preprocessed, i.e. converted from string to the required type. And how radically differently must a program be designed for this same problem if the data are entered from standard input (V2).
Text: in Italian only
Text attachment: temperature.go
Type: Program analysis
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. In particular the focus here is on passing data between main and a function (from main to a function through its parameters and from a function to main through returned values).
Text: in Italian only
Text attachment: crazy_hyphenation.go
The next exercise proposes a debugging task:
Type: Program debugging
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. In particular the focus here is whether storing the series of input values is necessary or not and, if not necessary, how many values are needed at a time.
Text: in English
Text attachment: sequence_int_bug.go
From now on, in the examples of execution we use the convention of representing the user input in bold font and the program output in monospace plain font.
The first of such exercises asks to modify a given program:
Type: Program tailoring
Topic: Input handling
Exercise purpose: The exercise helps reflect on typical issues concerning input handling. In particular the focus here is on reading single characters, which presents some issues in most languages.
Text: in English
Next we have a sequence of decreasingly scaffolded exercises that require writing a program.
At first, students are provided some preliminary observations derived from the analysis of the requirements specification, which makes the goals to be achieved explicit.
Type: Program writing from requirements specification guided by observations
Topic: Input handling
Exercise purpose: The exercise prepares the writing process with preliminary observations derived from the analysis of the requirements specification on typical issues concerning input handling: how is the input provided, what type are the values and if uniform (a series) or not, how must they be stored, when does the reading stop. Here the input is a series of integers ended by 0 from standard input.
Text: in Italian only
Type: program writing from requirements specifications guided by observations
Topic: Input handling
Exercise purpose: The exercise helps practice on typical issues concerning input handling and prepares the writing process with preliminary observations derived from the analysis of the requirements specification. Here the input is a series of strings from the command line.
Text: in Italian only
In the following questions there are no explicit observation; students instead are prompted some questions that prepare for the writing process.
Type: Program tailoring guided through questions
Topic: Input handling
Exercise purpose: The exercise helps practice on typical issues concerning input handling and prepares the writing process with questions that guide the analysis of the requirements specification. Here the input is a strings’ series of arbitrary length from standard input.
Text: In Italian only
Type: Program writing guided through questions
Topic: Input handling
Exercise purpose: The exercise helps practice on typical issues concerning input handling and prepares the writing process with questions that guide the analysis of the requirements specification. Here part of the data are passed between main and a function.
Text: in Italian only
Type: Program writing guided through questions
Topic: Input handling
Exercise purpose: The exercise helps practice on typical issues concerning input handling and prepares the writing process with questions that guide the analysis of the requirements specification. Here the input is a string from the command line, to be processed as a series of characters.
Text: in Italian
The last exercise asks to write a program from scratch, starting from requirements specifications written in natural language, with no further scaffolding.
Type: Program writing guided through questions
Topic: Input handling
Exercise purpose: The exercise helps practice on typical issues concerning input handling and prepares the writing process with questions that guide the analysis of the requirements specification. Here the input is a string from standard input, to be processed as a series of characters.
Text: in Italian