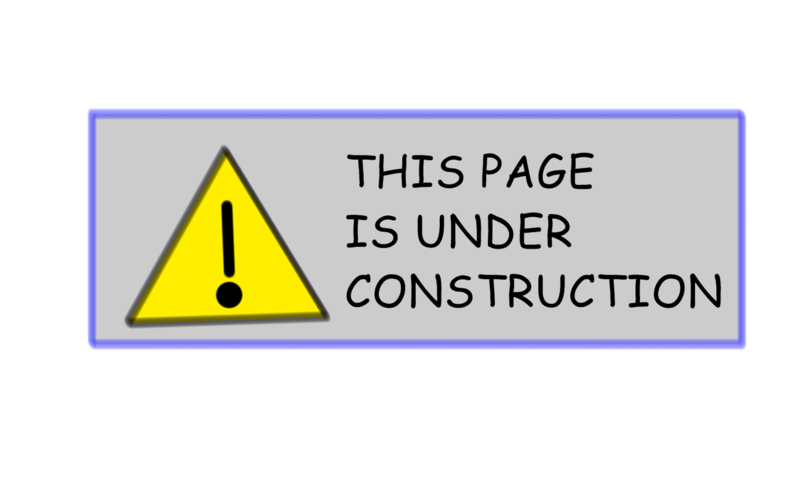
Capitals
Consider the following program capitals.go
that reads a line of text from standard input and outputs the line of text all capitalized.
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
var s string
const BASE_LOWER = ’a’
const BASE_UPPER = ’A’
:= bufio.NewScanner(os.Stdin)
scanner .Println("Enter a string to be converted to uppercase:")
fmtif scanner.Scan() {
= scanner.Text()
s }
.Println("The string's length is", len(s))
fmt
for _, char := range s {
if char >= ’a’ && char <= ’z’ {
:= char - BASE_LOWER
n = BASE_UPPER + n
char }
.Print(string(char))
fmt}
.Println()
fmt}
Analyze the source code and answer the following questions.
- Through which channel does the program receive the data to be processed?
- What type are the input data?
- Are the input data ready to be processed or are they first preprocessed in order to compute/extract other data?