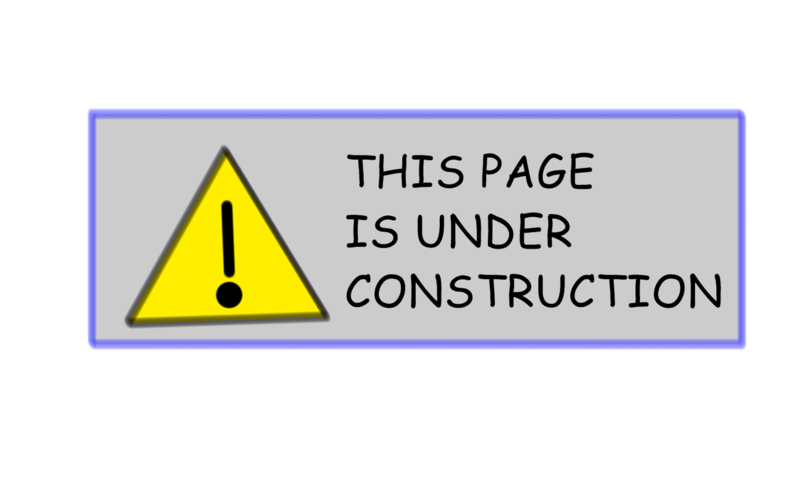
Exercise: Analysis of requirements specifications to identify goals
For each of the following requirements specifications, answer these questions:
- In the implementation of the program, which is the main goal to achieve?
- Which variables are needed in the implementation of the corresponding plan? How do they have to be initialized?
1. Solved example:
Requirements: Write a program that reads a sequence of words from standard input and prints how many of them contain the character ‘a’.
Answers:
- The main goal in this problem is a counting goal.
- To implement the corresponding plan, a Stepper variable is needed which keeps track of how many ‘a’’s have been encountered so far and which must be initialized to 0.
Requirements:
Write a function which, given a slice of integers as parameter, finds the ones that are greater than 7 and multiple of 4 and returns their product.
Write a program that reads 10 integers from standard input and for each one prints if it is even or odd.
Write a program that reads a sequence of words from standard input and prints the first word which begins the character ‘a’, or “no word with ‘a’ found”
Write a program that reads a sequence of integers from standard input and, for each one after the first one, prints if it is the successor of the number entered before it.
Write a function that receives a word as parameter and returns
true
if the word contains (at least) a double letter,false
otherwise.Write a program that reads a sequence of words from standard input and prints the length of the shortest word.
Write a program that reads an integer number from the command line. The number represents the balance of a bank account. The program then reads a sequence of expenses to be charged to the bank account and prints the final balance.
Write a program that reads a sequence of integers from standard input and prints the smallest odd number among them. The input sequence may contain both positive and negative numbers.
Write a program that reads a sequence of positive integers ended by -1 from standard input and prints the first number greater than 100, if there is one; otherwise it prints “no numbers greater than 100”.
Write a program that reads a sequence of positive integers ended by 0 from standard input, prints ’+’ every time the new value is greater than or equal to the preceding one, ‘-’ otherwise.